
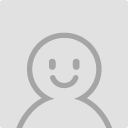
Add code for DIP. Update README.md
@34dda4f85d8e4c686e9e0f342ec8533c7305edc5
--- README.md
+++ README.md
... | ... | @@ -1,7 +1,7 @@ |
1 | 1 |
## SOLID - Principles that Apply |
2 | 2 |
|
3 | 3 |
This page is destinated to collect some good resources (IMHO) in order to give |
4 |
-an overview over good priciples that should apply to software designe. |
|
4 |
+an overview over good priciples that should be applied to software design. |
|
5 | 5 |
|
6 | 6 |
- [Intro to `SOLID`](http://www.wikiwand.com/en/SOLID_(object-oriented_design)) |
7 | 7 |
- `S`: Single Responsibility Principle. |
... | ... | @@ -17,16 +17,21 @@ |
17 | 17 |
- `I`: Interface Segregation Principle |
18 | 18 |
- [link](http://www.oodesign.com/dependency-inversion-principle.html) |
19 | 19 |
- [Wiki](https://en.wikipedia.org/wiki/Interface_segregation_principle) |
20 |
- - `D`: |
|
20 |
+ - `D`: Dependency Inversion Principle |
|
21 |
+ - [link](http://www.oodesign.com/dependency-inversion-principle.html) |
|
22 |
+ - [Code Tutorials](http://code.tutsplus.com/tutorials/solid-part-4-the-dependency-inversion-principle--net-36872) |
|
23 |
+ - **Program to an interface, not an implementation.** |
|
21 | 24 |
|
22 | 25 |
## Running the Code |
23 | 26 |
|
24 | 27 |
All the submodules are managed in the same way, which means you can run the code using the same syntax. For example, if you want to see the result of the sample code for Open/Close principle, just run: |
25 | 28 |
|
26 | 29 |
``` |
27 |
-python -m python_code.bad.open\_close # See the result of bad implementation |
|
28 |
-python -m python_code.good.open\_close # See the result of good implementation |
|
30 |
+python -m python_code.bad.open_close # See the result of bad implementationgi |
|
31 |
+python -m python_code.good.open_close # See the result of good implementation |
|
29 | 32 |
``` |
33 |
+ |
|
34 |
+Or, you can run `python -m python_code --list` or `python -m python_code -l` to see all the available submodules. |
|
30 | 35 |
|
31 | 36 |
However, it is recommanded to see the source code and get an understanding of what the issue is and possible way to solve it. |
32 | 37 |
|
... | ... | @@ -37,3 +42,5 @@ |
37 | 42 |
- [Programming Done By Superstition](https://utcc.utoronto.ca/~cks/space/blog/programming/ProgrammingViaSuperstition) |
38 | 43 |
- [Object Mentor](http://www.objectmentor.com/resources/publishedArticles.html) |
39 | 44 |
- [Clean Coder](http://cleancoders.com/category/fundamentals) |
45 |
+- [Code Tutorials](http://code.tutsplus.com/series/the-solid-principles--cms-634) |
|
46 |
+- [搞笑談軟工](http://teddy-chen-tw.blogspot.tw/2012/01/5dependency-inversion-principle.html) |
+++ python_code/__main__.py
... | ... | @@ -0,0 +1,15 @@ |
1 | +#!/usr/bin/env python | |
2 | +# -*- coding: utf-8 -*- | |
3 | + | |
4 | +from good import __all__ as all_submodules | |
5 | +import sys | |
6 | + | |
7 | +def main(argv): | |
8 | + if len(argv) > 1 and argv[1] in ["-l", "--list"]: | |
9 | + print "Available submodules: " | |
10 | + for i, submodule in enumerate(all_submodules, 1): | |
11 | + print "{}. good.{} / bad.{}".format(i, submodule, submodule) | |
12 | + | |
13 | +if __name__ == "__main__": | |
14 | + | |
15 | + main(sys.argv)(파일 끝에 줄바꿈 문자 없음) |
+++ python_code/bad/DIP.py
... | ... | @@ -0,0 +1,50 @@ |
1 | +#!/usr/bin/env python | |
2 | +# -*- coding: utf-8 -*- | |
3 | + | |
4 | +class Worker(object): | |
5 | + | |
6 | + def work(self): | |
7 | + print "I'm working!!" | |
8 | + | |
9 | + | |
10 | +class Manager(object): | |
11 | + | |
12 | + def __init__(self): | |
13 | + self.worker = None | |
14 | + | |
15 | + def set_worker(self, worker): | |
16 | + assert isinstance(worker, Worker), '`worker` must be of type {}'.format(Worker) | |
17 | + | |
18 | + self.worker = worker | |
19 | + | |
20 | + def manage(self): | |
21 | + if self.worker is not None: | |
22 | + self.worker.work() | |
23 | + # And some complex codes go here.... | |
24 | + | |
25 | +class SuperWorker(object): | |
26 | + | |
27 | + def work(self): | |
28 | + print "I work very hard!!!" | |
29 | + | |
30 | +# OK... now you can see what happend if we want the `Manager` to support `SuperWorker`. | |
31 | +# 1. The `set_worker` must be modified or it will not pass the type-checking. | |
32 | +# 2. The `manage` method should be re-test, which means you may or may not have to | |
33 | +# rewrite the testing code. | |
34 | + | |
35 | +def main(): | |
36 | + | |
37 | + worker = Worker() | |
38 | + manager = Manager() | |
39 | + manager.set_worker(worker) | |
40 | + manager.manage() | |
41 | + | |
42 | + # The following will not work... | |
43 | + super_worker = SuperWorker() | |
44 | + try: | |
45 | + manager.set_worker(super_worker) | |
46 | + except AssertionError: | |
47 | + print "manager fails to support super_worker...." | |
48 | + | |
49 | +if __name__ == "__main__": | |
50 | + main() |
--- python_code/bad/__init__.py
+++ python_code/bad/__init__.py
... | ... | @@ -1,0 +1,4 @@ |
1 |
+#/usr/bin/env python |
|
2 |
+# -*- coding: utf-8 -*- |
|
3 |
+ |
|
4 |
+__all__ = ['DIP', 'ISP', 'LSP', 'open_close', 'single_responsibility'](파일 끝에 줄바꿈 문자 없음) |
+++ python_code/good/DIP.py
... | ... | @@ -0,0 +1,63 @@ |
1 | +#!/usr/bin/env python | |
2 | +# -*- coding: utf-8 -*- | |
3 | + | |
4 | +# Here we solve the issues in the design in `python_code.bad.DIP` with an interface | |
5 | +# (implemented with abstract class). | |
6 | + | |
7 | +from abc import ABCMeta, abstractmethod | |
8 | + | |
9 | +class IWorker(object): | |
10 | + __metaclass__ = ABCMeta | |
11 | + | |
12 | + @abstractmethod | |
13 | + def work(self): | |
14 | + pass | |
15 | + | |
16 | +# `IWorker` defines a interface which requires `work` method. | |
17 | + | |
18 | +class Worker(IWorker): | |
19 | + | |
20 | + def work(self): | |
21 | + print "I'm working!!" | |
22 | + | |
23 | + | |
24 | +class Manager(object): | |
25 | + | |
26 | + def __init__(self): | |
27 | + self.worker = None | |
28 | + | |
29 | + def set_worker(self, worker): | |
30 | + assert isinstance(worker, IWorker), '`worker` must be of type {}'.format(Worker) | |
31 | + | |
32 | + self.worker = worker | |
33 | + | |
34 | + def manage(self): | |
35 | + if self.worker is not None: | |
36 | + self.worker.work() | |
37 | + # And some complex codes go here.... | |
38 | + | |
39 | +class SuperWorker(IWorker): | |
40 | + | |
41 | + def work(self): | |
42 | + print "I work very hard!!!" | |
43 | + | |
44 | +# Now, the manager support `SuperWorker`... | |
45 | +# In addition, it will support any worker which obeys the interface defined by `IWorker`! | |
46 | + | |
47 | +def main(): | |
48 | + | |
49 | + worker = Worker() | |
50 | + manager = Manager() | |
51 | + manager.set_worker(worker) | |
52 | + manager.manage() | |
53 | + | |
54 | + # The following will not work... | |
55 | + super_worker = SuperWorker() | |
56 | + try: | |
57 | + manager.set_worker(super_worker) | |
58 | + manager.manage() | |
59 | + except AssertionError: | |
60 | + print "manager fails to support super_worker...." | |
61 | + | |
62 | +if __name__ == "__main__": | |
63 | + main() |
--- python_code/good/__init__.py
+++ python_code/good/__init__.py
... | ... | @@ -1,0 +1,4 @@ |
1 |
+#/usr/bin/env python |
|
2 |
+# -*- coding: utf-8 -*- |
|
3 |
+ |
|
4 |
+__all__ = ['DIP', 'ISP', 'LSP', 'open_close', 'single_responsibility'](파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?